Notice
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- 달력 라이브러리
- 캘린더 라이브러리
- ui탬플릿
- 배포
- RCPS
- 관리자페이지
- Ajax
- 로그인 로직
- 마이페이지
- Update
- 로그인
- register
- jsonwebtoken
- 스프링시큐리티
- Styled Components
- MRC
- Token
- 파생상품평가
- 밸류즈 홈페이지
- 밸류즈
- stock option
- 인증처리
- 회원가입로직
- userManagement
- Typesciprt
- 이미지 업로드
- react
- mypage
- 빌드 및 배포
- 공통메서드
Archives
- Today
- Total
I T H
[MySchedule project] 3. frontend - redux 세팅(redux-toolkit사용) + typescript 본문
React + Node.js
[MySchedule project] 3. frontend - redux 세팅(redux-toolkit사용) + typescript
thdev 2024. 1. 25. 10:56이번시간에는 상태관리 라이브러리인 리덕스를 사용해보겠다.
tsx파일에서의 리덕스 적용과 리덕스를 효율적이고 복잡하지 않게 로직을 짤수있는 라이브러리인 redux-toolkit을 사용할 예정.
- 리덕스 툴킷을 사용하면 createSlice를 통해 action과 reducer를 효과적으로 사용할 수 있다.
흐름: ui -> action -> reducer -> store -> ui 사이클이 형성된다.
Redux hooks의 기술
- useDispatch : action을 생성해준다. 즉 버튼클릭과 같은 이벤트 실행시 reducer를 호출하고 reducer는 새로운 store를 생성함.
action 객체가 바로 store에 전달되는것이 아닌 reducer를 통해 store의 상태값을 변환시켜주는 것임.
reducer를 통해 전달하기 위해선 바로 useDispatch가 필요한 것이다.
- useSelector : 스토어에 있는 상태값을 가져온다.
정리 : action -> useDispatch(action에서 reducer호출) -> reducer -> store안의 state(상태값)을 업데이트 -> ui
* 이번 챕터는 존안님의 강의와 리덕스툴킷_typescript 공식문서 + 별도의 구글링을 참고해서 작성하였음.
[ store폴더 / redux.ts]
- 가독성을 위해 리덕스와 관련된 타입들을 모아논 ts 파일이다.
- useSlice나 useDispatch함수들의 타입을 미리 지정해놓고 import 해서 사용할 예정.
import { TypedUseSelectorHook, useDispatch, useSelector } from "react-redux";
import { store } from "./index";
// 타입스크립트 적용 hook start
export type RootState = ReturnType<typeof store.getState>;
//export type AddDispatch = typeof store.dispatch;
//export const useAddDispatch = () => useDispatch<AddDispatch>();
export const useAddDispatch = () => useDispatch<typeof store.dispatch>();
export const useAddSelector: TypedUseSelectorHook<RootState> = useSelector;
// 타입스크립트 적용 hook end
export interface User {
userData: basicUser;
isAuth: boolean;
isLoading: boolean;
error: string;
}
export interface basicUser {
userId: string;
userName: string;
userPhone: string;
userEmail: string;
userImage: string;
role: number;
}
[ store폴더 / index.tsx ]
store 의 reducer를 설정할때 중요한 포인트 두가지를 먼저 살펴보겠다.
- redux persist : 리덕스의 상태를 지속적으로 유지하기위한 라이브러리로 세션 종료나 페이지 새로고침시에도 상태가 유지됨.
- combineReducers : 값이 다른 리듀서 함수들을 하나의 리듀싱함수로 합쳐주는 역할
import { combineReducers, configureStore } from "@reduxjs/toolkit";
import userReducer from "./userSlice";
import storage from "redux-persist/lib/storage";
import {
FLUSH,
PAUSE,
PERSIST,
PURGE,
REGISTER,
REHYDRATE,
persistReducer,
persistStore,
} from "redux-persist";
const rootReducer = combineReducers({
//여러 리듀서들을 합쳐준다.
user: userReducer,
});
//persist : 지속하다. //redux의 state 즉 상태값들을 지속적으로 유지시켜준다.
const persistConfig = {
key: "root", //key이름
storage, //localStorage에 저장합니다.
//whitelist: [ ] 여러 reducer중에 해당 reducer만 localStorage에 저장
//blacklist: [] 그것만 제외
};
const persistedReducer = persistReducer(persistConfig, rootReducer);
export const store = configureStore({
reducer: persistedReducer,
middleware: (getDefaultMiddleware) =>
getDefaultMiddleware({
serializableCheck: {
//serializable == 직렬화 : OBJECT -> STRING 으로 변환
//리덕스는 직렬화 되지 않은 값들은 에러로 처리하기 때문에 serializableCheck를 ignored 한다.(false)
ignoredActions: [FLUSH, REHYDRATE, PAUSE, PERSIST, PURGE, REGISTER],
},
}),
});
export const persistor = persistStore(store);
[store폴더 - userSlice.tsx]
- initialState를 통해 초기값을 먼저 설정하고, userSlice를 만들어주었다.
- tsx 파일이기에 initialState의 초기값에 대한 타입도 지정해두어야한다.
위에 지정해놓은 redux.ts파일에서 가져와서 사용.
- userSlice의 reducer는 store의 index.js에서 combineReducers를 통해 reducer들을 합쳐준다.
import { createSlice } from "@reduxjs/toolkit";
import { userRegister } from "./thunkFunction";
import { toast } from "react-toastify";
import { User } from "./redux";
const initialState: User = {
userData: {
userId: "", //사용자 아이디
userName: "",
userPhone: "",
userEmail: "",
userImage: "",
role: 0, //권한 : 일반사용자 0, 관리자 1
},
isAuth: false,
isLoading: false,
error: "",
};
const userSlice = createSlice({
name: "user",
initialState: initialState,
reducers: {},
extraReducers: (builder) => {
},
});
export default userSlice.reducer;
[index.tsx]
- store 기본 세팅이 되었으면 <App>컴포넌트를 <Provider>로 감싸서 store를 넣어주고, <persistGate>로 감싸서 리덕스 스토어의 상태값들을 지속시켜준다.
import React from "react";
import ReactDOM from "react-dom/client";
import App from "./App.jsx";
import "./index.css";
import { BrowserRouter } from "react-router-dom";
import { Provider } from "react-redux";
import { persistor, store } from "./store/index";
import { PersistGate } from "redux-persist/integration/react";
ReactDOM.createRoot(document.getElementById("root")).render(
<BrowserRouter>
<Provider store={store}>
<PersistGate loading={null} persistor={persistor}>
<App />
</PersistGate>
</Provider>
</BrowserRouter>
);
[결과화면]
- 리덕스 세팅이 완료되었으면 개발자도구에서 state상태가 다음과 같이 만들어졌으면 정상작동!
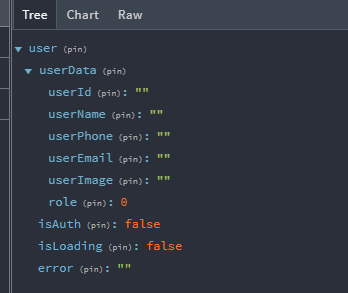
'React + Node.js' 카테고리의 다른 글
[MySchedule project] 6. JWT(json web token) / 로그인 로직(1) (2) | 2024.01.30 |
---|---|
[MySchedule project] 5. backend - 회원가입 로직 (0) | 2024.01.25 |
[MySchedule project] 4. frontend - 회원가입 로직 (1) | 2024.01.25 |
[MySchedule project] 2. frontend - 레이아웃 / react- router 설정 (1) | 2024.01.25 |
[MySchedule project] 1. frontend - 타입스크립트 초기 환경설정 (0) | 2024.01.25 |